I have already written several posts regarding Android database applications. This post might be similar to those tuts. However this is more complete Android Login System which uses SQLite as its database. All files can be downloaded here or there
Let's create the view. first. But I'm not going to show it in the post. Just two text boxes for username and password, two buttons for login and register.
DBHelper.java
[sourcecode language="java"]
package com.my.members;
import android.content.Context;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
public class DBHelper extends SQLiteOpenHelper {
private static final String DATABASE_NAME = "membersdb";
private static final int DATABASE_VERSION = 1;
private static final String DATABASE_CREATE = "CREATE TABLE (_id integer primary key autoincrement,username text not null,password text not null);";
public DBHelper(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL(DATABASE_CREATE);
}
@Override
public void onUpgrade(SQLiteDatabase db, int arg1, int arg2) {
db.execSQL("DROP TABLE IF EXISTS members");
onCreate(db);
}
}
[/sourcecode]
DBAdapter.java
[sourcecode language="java"]
package com.my.members;
import android.content.ContentValues;
import android.content.Context;
import android.database.Cursor;
import android.database.SQLException;
import android.database.sqlite.SQLiteDatabase;
public class DBAdapter
{
private static final String DATABASE_TABLE = "members";
public static final String KEY_ROW_ID = "_id";
public static final String KEY_USERNAME = "username";
public static final String KEY_PASSWORD = "password";
SQLiteDatabase mDb;
Context mCtx;
DBHelper mDbHelper;
public DBAdapter(Context context)
{
this.mCtx = context;
}
public DBAdapter open() throws SQLException
{
mDbHelper = new DBHelper(mCtx);
mDb = mDbHelper.getWritableDatabase();
return this;
}
public void close()
{
mDbHelper.close();
}
public long register(String user,String pw)
{
ContentValues initialValues = new ContentValues();
initialValues.put(KEY_USERNAME, user);
initialValues.put(KEY_PASSWORD, pw);
return mDb.insert(DATABASE_TABLE, null, initialValues);
}
public boolean Login(String username, String password) throws SQLException
{
Cursor mCursor = mDb.rawQuery("SELECT * FROM " + DATABASE_TABLE + " WHERE username=? AND password=?", new String[]{username,password});
if (mCursor != null) {
if(mCursor.getCount() > 0)
{
return true;
}
}
return false;
}
}
[/sourcecode]
MembersActivity
[sourcecode language="java"]
package com.my.members;
import android.app.Activity;
import android.content.Context;
import android.database.SQLException;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.view.View.OnClickListener;
import android.view.inputmethod.InputMethodManager;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MembersActivity extends Activity {
DBAdapter dbAdapter;
EditText txtUserName;
EditText txtPassword;
Button btnLogin;
Button btnRegister;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
txtUserName = (EditText) findViewById(R.id.et_user);
txtPassword = (EditText) findViewById(R.id.et_pw);
btnLogin = (Button) findViewById(R.id.btn_login);
btnRegister = (Button) findViewById(R.id.btn_reg);
dbAdapter = new DBAdapter(this);
dbAdapter.open();
btnLogin.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
InputMethodManager imm = (InputMethodManager) getSystemService(Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(txtUserName.getWindowToken(), 0);
imm.hideSoftInputFromWindow(txtPassword.getWindowToken(), 0);
String username = txtUserName.getText().toString();
String password = txtPassword.getText().toString();
if (username.length() > 0 && password.length() > 0) {
try {
if (dbAdapter.Login(username, password)) {
Toast.makeText(MembersActivity.this,
"Successfully Logged In", Toast.LENGTH_LONG)
.show();
} else {
Toast.makeText(MembersActivity.this,
"Invalid username or password",
Toast.LENGTH_LONG).show();
}
} catch (Exception e) {
Toast.makeText(MembersActivity.this, "Some problem occurred",
Toast.LENGTH_LONG).show();
}
} else {
Toast.makeText(MembersActivity.this,
"Username or Password is empty", Toast.LENGTH_LONG).show();
}
}
});
btnRegister.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
InputMethodManager imm = (InputMethodManager) getSystemService(Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(txtUserName.getWindowToken(), 0);
imm.hideSoftInputFromWindow(txtPassword.getWindowToken(), 0);
try {
String username = txtUserName.getText().toString();
String password = txtPassword.getText().toString();
long i = dbAdapter.register(username, password);
if(i != -1)
Toast.makeText(MembersActivity.this, "You have successfully registered",Toast.LENGTH_LONG).show();
} catch (SQLException e) {
Toast.makeText(MembersActivity.this, "Some problem occurred",
Toast.LENGTH_LONG).show();
}
}
});
}
}
[/sourcecode]
Donate & help live a developer
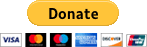
Please tell me what is the use of these statements
ReplyDeleteInputMethodManager imm = (InputMethodManager) getSystemService(Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(txtUserName.getWindowToken(), 0);
imm.hideSoftInputFromWindow(txtPassword.getWindowToken(), 0);
Hi Vivek,
ReplyDeleteInputMethodManager basically controls the interaction between application and current input method. This happens in client side and within application context. You better try reading Android reference.
http://developer.android.com/reference/android/view/inputmethod/InputMethodManager.html
Thank you so so so so so Much dear friends.....
ReplyDeleteHi Qutub,
ReplyDeleteThanks for commenting.
Sir, I trying this project but, i get error on.
ReplyDeletethe message android.database.sqlite.SQLiteException: near "(": syntax error: CREATE TABLE
(_id integer primary key autoincrement,username text not null,password text not null)
please tell me, where is the part make it error i'm newbe in android project
thanks
Can you cpoy & paste the errorneous piece of code here?
ReplyDeletei just download it from the complete source, and then i run it, but error. the message android.database.sqlite.SQLiteException: near “(“: syntax error: CREATE TABLE
ReplyDelete(_id integer primary key autoincrement,username text not null,password text not null)
am get error on syntax error: CREATE TABLE(..........)
ReplyDeleteHelp me
Ayas, try this code sure it will work
ReplyDeleteprivate static final String DATABASE_TABLE = "users";
private static final String DATABASE_CREATE = "create table users (_id integer primary key autoincrement, "
+ "username text not null, "
+ "password text not null);";
Help me!!!
ReplyDeletememberactivity get me error :: The method register(String, String) is undefined for the type DBAdapter!
i trying to create two dbtable in my app but problem is that 2nd table did't created...
ReplyDeletemy logcat error:
03-03 02:13:51.402: E/SQLiteLog(1473): (1) no such table: TRADE
plz help me if anybody know this:
Hi,
ReplyDeleteDon't know whether you solved that.
Please find this function.
public void onCreate(SQLiteDatabase db) {
db.execSQL(DATABASE_CREATE);
}
When the function is executed, members table is created according to the example.
private static final String DATABASE_CREATE = "CREATE TABLE (_id integer primary key autoincrement,username text not null,password text not null);";
If your first table is created, then you may need to
update database version
Eg. private static final int DATABASE_VERSION = 2;.
Please La Aplication Dowmload.. Help Me!!
ReplyDeleteunfortunately stop of application is occuring when i am running the code???
ReplyDeleteThanks for the help, just a couple of questions:
ReplyDeleteWhat's the use of context? I just want to get that part clear.
Thanks again for your help
Hi,
ReplyDeleteContext is simply the current state of the application/object. You can get the context by invoking getApplicationContext(), getContext(), getBaseContext() or this (when in the activity class).
Example usage:
TextView tv = new TextView(getContext());
ListAdapter adapter = new SimpleCursorAdapter(getApplicationContext(), ...);
Its an entity that represents various environment data . Context helps the current activity to interact with out side android environment like local files, databases, class loaders associated to the environment, services including system-level services, and more.
How do I log out a user using this approach?
ReplyDeletehow do i get to a new activity after a successful login
ReplyDeleteplease use .zip format for source code distribution, as because .zip can be open in windows/mac/ubuntu without installing additional software. while Members.rar format will need to install additional software in all these platforms.
ReplyDeleteThanks Abhishek for your point. Yes, this is a mistake. I'll upload .zip file now.
ReplyDeleteMUCHAS GRACIAS me sirvió el LOGIN PERFECTO
ReplyDeletegracias por compartir tu conocimiento en android
THANK YOU SO MUCH. i do some modification for strings and class name but it not a big deal for my running login system. <3
ReplyDeleteHi, I would like to know if this would work in different devices. For example, if I register in a device, could I access with that account to the app from another device without registering again?
ReplyDeleteit shows unfortunately stopped
ReplyDeleteyou need the name of the table. Here it's members
ReplyDeleteHi i tried working this entire project ... there is no error but the "application stops unfortunately "
ReplyDeleteCould you please help me out
It shpws unfortunately stopped ... no error in the entire project
ReplyDeleteIt displays unfortunately stopped
ReplyDeleteno layout R.name.xml ?????????????????????????????????????
ReplyDeletehow to get the username and password from register tables using android studio and download app sir
ReplyDelete